Create an API Resource in API Platform
31 May 2022 (Updated 1 June 2022)
1. Generate an entity and mark it as an API resource
If you haven’t already, install the maker bundle with symfony composer req maker
.
Generate an entity:
./bin/console make:entity
Give it a name (e.g., CheeseListing
).
When asked, choose to mark the class an API Platform resource.
This will create a src/Entity/CheeseListing.php
file that looks something like:
<?php
namespace App\Entity;
use ApiPlatform\Core\Annotation\ApiResource;
use App\Repository\CheeseListingRepository;
use Doctrine\ORM\Mapping as ORM;
#[ORM\Entity(repositoryClass: CheeseListingRepository::class)]
#[ApiResource]
class CheeseListing
{
#[ORM\Id]
#[ORM\GeneratedValue]
#[ORM\Column(type: 'integer')]
private $id;
public function getId(): ?int
{
return $this->id;
}
}
Marking the entity as an ApiResource adds the #[ApiResource]
annotation.
2. Generate and run migration
Generate migration:
./bin/console make:migration
Check generated migration to ensure it’s what you want and then run:
./bin/console doc:mig:mig
3. Browse Swagger docs for new resource
Visit /api
and you should see the endpoints for your new resource:
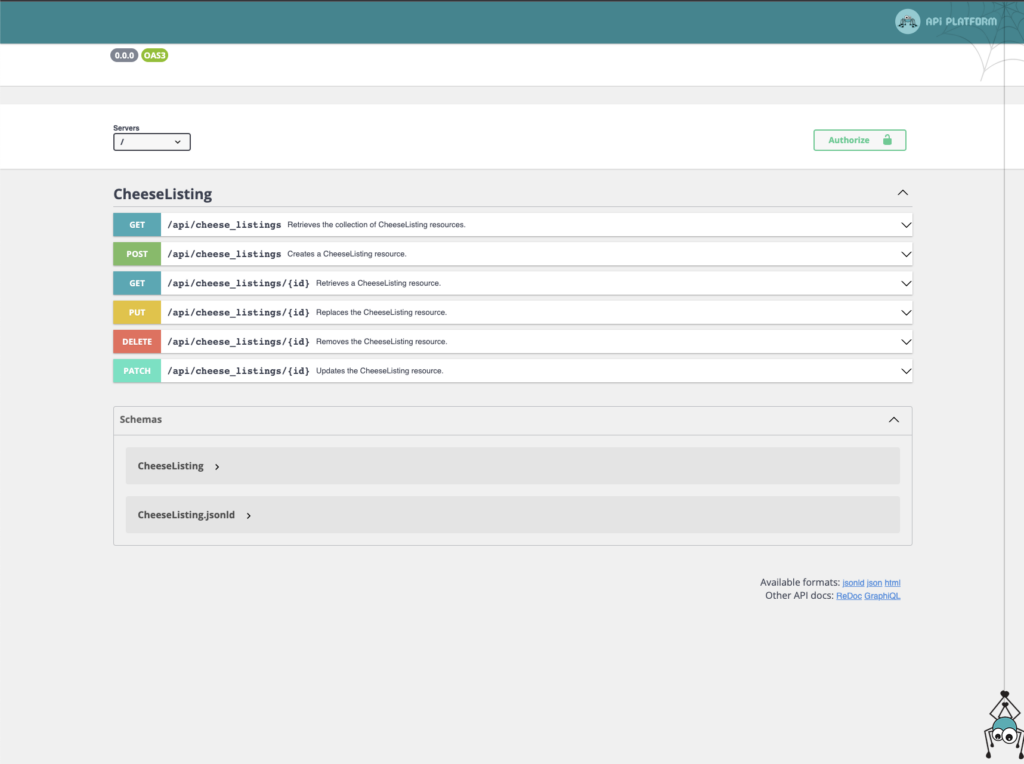
You can also view the routes for the resource with:
./bin/console debug:router
Example output:
------------------------------------- -------- -------- ------ -------------------------------------
Name Method Scheme Host Path
------------------------------------- -------- -------- ------ -------------------------------------
api_entrypoint ANY ANY ANY /api/{index}.{_format}
api_doc ANY ANY ANY /api/docs.{_format}
api_jsonld_context ANY ANY ANY /api/contexts/{shortName}.{_format}
api_cheese_listings_get_collection GET ANY ANY /api/cheese_listings.{_format}
api_cheese_listings_post_collection POST ANY ANY /api/cheese_listings.{_format}
api_cheese_listings_get_item GET ANY ANY /api/cheese_listings/{id}.{_format}
api_cheese_listings_delete_item DELETE ANY ANY /api/cheese_listings/{id}.{_format}
api_cheese_listings_put_item PUT ANY ANY /api/cheese_listings/{id}.{_format}
api_cheese_listings_patch_item PATCH ANY ANY /api/cheese_listings/{id}.{_format}
_preview_error ANY ANY ANY /_error/{code}.{_format}
------------------------------------- -------- -------- ------ -------------------------------------
Tagged:
API Platform
Thanks for your comment 🙏. Once it's approved, it will appear here.
Leave a comment