Dump helper component for React
17 April 2022 (Updated 19 September 2022)
Sometimes, you want to see a dump of some data in React, ideally in a pretty format. Here’s a little component that can help:
import React, { useState } from 'react'
interface Props {
title: string;
value: any;
expanded?: boolean
}
const Dump: React.FC<Props> = ({ title, value, expanded = false }) => {
const [isExpanded, setIsExpanded] = useState<boolean>(expanded)
const toggleValues = () => setIsExpanded(!isExpanded)
return (
<div
style={{
backgroundColor: "#f5f5f5",
margin: "15px 0",
padding: "12px",
}}
>
<div
style={{ alignItems: 'center', cursor: 'pointer', display: 'flex' }}
onClick={toggleValues}
>
{title}
<span style={{ color: '#393939', fontSize: '10px', marginLeft: '7px' }}>
{isExpanded ? '▲' : '▼'}
</span>
</div>
{isExpanded && (
<pre style={{ marginTop: '10px' }}>
{JSON.stringify(value, null, 2)}
</pre>
)}
</div>
)
}
export default Dump
Now, you can use it like this:
<Dump values={myFormState} title="formValues" />
If you want the dump data to be expanded by default, add the expanded
attribute:
<Dump values={myFormState} title="formValues" expanded />
This should give you something like this:
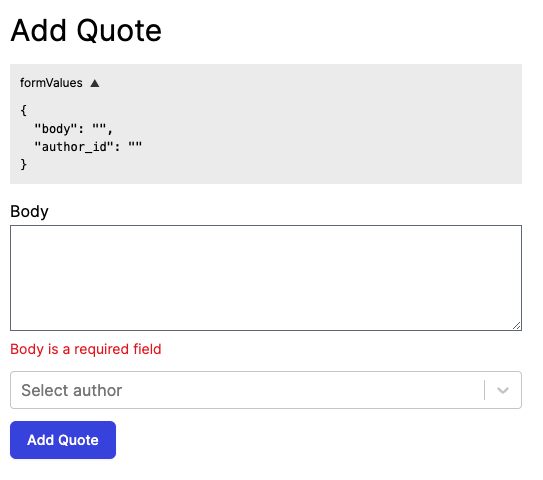
Tailwind version
import React, { useState } from 'react'
interface Props {
title: string
value: any
expanded?: boolean
}
const Dump: React.FC<Props> = ({ title, value, expanded = false }) => {
const [isExpanded, setIsExpanded] = useState<boolean>(expanded)
const toggleValues = () => setIsExpanded(!isExpanded)
return (
<div className="bg-gray-100 text-sm mt-4 p-3">
<div className="flex items-center cursor-pointer" onClick={toggleValues}>
{title}
<span className="text-gray-800 font-[10px] ml-1.5">
{isExpanded ? '▲' : '▼'}
</span>
</div>
{isExpanded && (
<pre className="mt-2.5">{JSON.stringify(value, null, 2)}</pre>
)}
</div>
)
}
export default Dump
Tagged:
React tooling