How to view request data inside a Form class
1 September 2024 (Updated 1 September 2024)
Suppose you have a StorePersonRequest
class like this:
And a PersonController#store
method that uses that request:
How can you inspect the incoming data inside the request class?
You can either add a breakpoint in the request class’s authorize()
method and check $this->input()
or you can do a dump($this->input()
or dd($this->input())
:
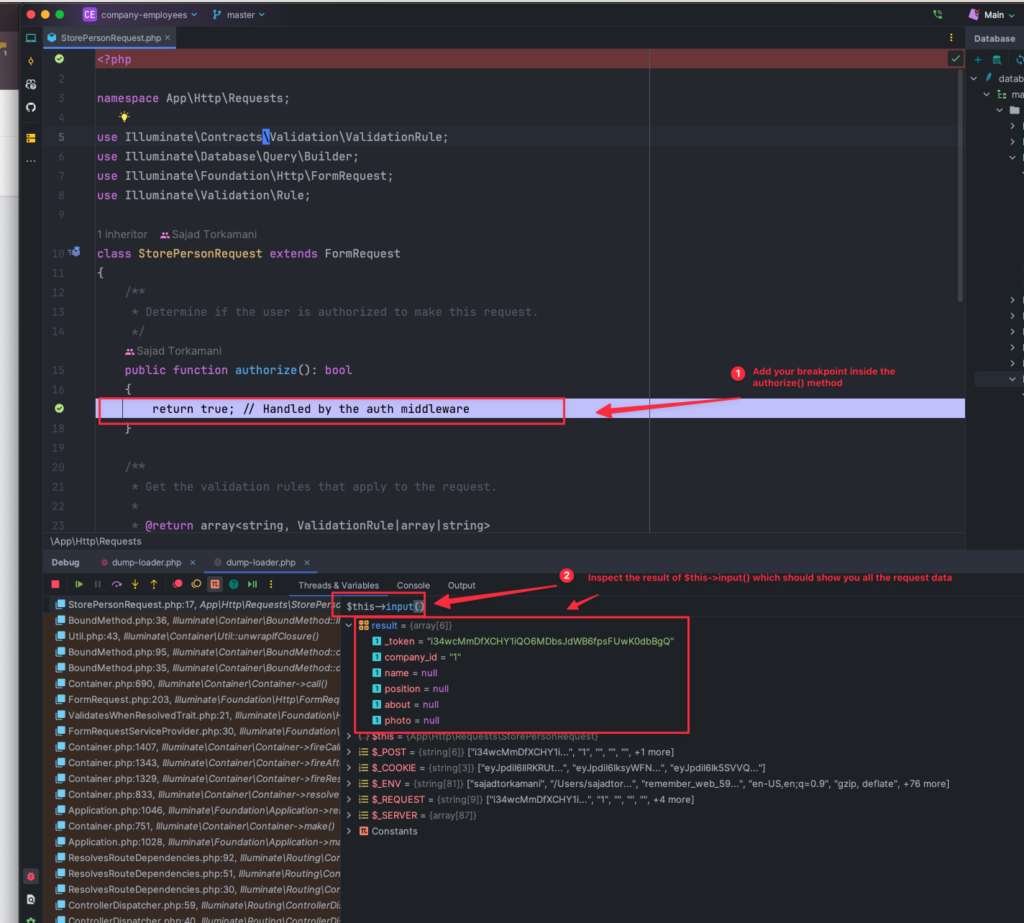
Tagged:
Laravel