Serialize PHP objects using Symfony Serializer
2 June 2022 (Updated 6 June 2022)
What is serialization?
Serialization is the process of converting a PHP object into a data format like JSON or XML. In PHP, It requires first normalizing the object into an array and then encoding the array into the target format. Serialization is the opposite of deserialization.
Serialization plays an essential part in building web services / REST APIs because you must be able to convert all sorts of incoming HTTP data (usually in JSON) to application-specific data types.
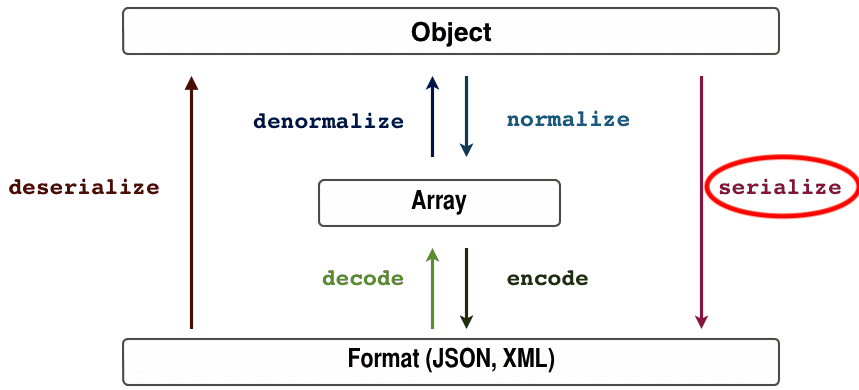
How to serialize
Install dependencies
Install the Serializer component:
composer require symfony/serializer
Assuming you’ll use the ObjectNormalizer
as your normalizer, install the PropertyAccess
component that it depends on:
composer require symfony/property-access
Define entities
Now, if assuming you have a standard PHP entity class:
<?php
// src/Entity/Person.php
namespace App\Entity;
use DateTimeImmutable;
class Person
{
private string $age;
private string $name;
private bool $isAlive;
private DateTimeImmutable $createdAt;
// Getters
public function getName(): string
{
return $this->name;
}
public function getAge(): string
{
return $this->age;
}
public function getCreatedAt(): DateTimeImmutable
{
return $this->createdAt;
}
// Issers
public function isAlive(): bool
{
return $this->isAlive;
}
// Setters
public function setName(string $name): void
{
$this->name = $name;
}
public function setAge(int $age): void
{
$this->age = $age;
}
public function setIsAlive(bool $isAlive): void
{
$this->isAlive = $isAlive;
}
public function setCreatedAt(DateTimeImmutable $createdAt): void
{
$this->createdAt = $createdAt;
}
}
Call the serializer
You can serialize it into a data format like JSON:
<?php
// src/serialize.php
use App\Entity\Person;
use Symfony\Component\Serializer\Encoder\JsonEncoder;
use Symfony\Component\Serializer\Encoder\XmlEncoder;
use Symfony\Component\Serializer\Normalizer\ObjectNormalizer;
use Symfony\Component\Serializer\Serializer;
require 'vendor/autoload.php';
$encoders = [new XmlEncoder(), new JsonEncoder()];
$normalizers = [new ObjectNormalizer()];
$serializer = new Serializer($normalizers, $encoders);
$person = new Person();
$person->setName('Sajad');
$person->setAge(28);
$person->setIsAlive(true);
$jsonContent = $serializer->serialize($person, 'json');
dump($jsonContent);
Running this should output:
"{"name":"Sajad","age":"28","alive":true}"
Sources
Tagged:
Symfony