How to publish an NPM package
An example package that we will publish
Let’s see what we need to do to publish a very small NPM package which once published should have a working README
that looks like this:
## Install
npm install funny-joke
### Usage
### Use as ESM module
import funnyJoke from 'funny-joke'
### Use as CommonJS module
const funnyJoke = require('funny-joke')
### Use in browser from a script tag
<script src="https://cdn.js.../path-to-package"></script>
<script>
console.log(window.funnyJoke())
</script>
See below for the GitHub repo and the published NPM package:
Ok, let’s get into it.
Create your module
Create an empty project folder and add a src/main.ts
file:
export default function funnyJoke() {
return "Why don't skeletons fight each other? They don't have the guts."
}
Setup remote Git repo
git init
git remote add origin git://git-remote-url # You'll need to create a repo on GitHub
Setup package.json
Copy and paste the following and modify as needed:
{
"name": "funny-joke",
"version": "0.0.1",
"description": "Get a funny joke",
"repository": {
"type": "git",
"url": "git+https://github.com/sajadtorkamani/funny-joke.git"
},
"author": "Sajad Torkamani <sajadtorkamani1@gmail.com>",
"license": "MIT",
"bugs": {
"url": "https://github.com/sajadtorkamani/funny-joke/issues"
},
"homepage": "https://github.com/sajadtorkamani/funny-joke#readme",
"main": "dist/funny-joke.cjs.js",
"module": "dist/funny-joke.esm.js",
"browser": "dist/funny-joke.umd.js",
"devDependencies": {
"prettier": "^3.0.3",
"rollup": "^4.1.1"
},
"scripts": {
"build": "rollup -c --bundleConfigAsCjs",
"dev": "rollup -c -w --bundleConfigAsCjs"
},
"files": [
"dist"
]
}
Run npm install
to install the dependencies.
Important fields to note:
files
: Specify which files you want to be included in thenode_modules
directory of the user when they runnpm install <your-package>
. Usually, this will be thedist
folder where you output the build files.main
: What is this?module
: Specifies the path tobrowser
: The UMD file (why is this needed? Is it for CDNs like unpkg?)repository
: The path to your GitHub repo. NPM will automatically link to this page.
See package.json reference for more on what these fields mean.
Create rollup.config.js
import pkg from './package.json'
export default [
// browser-friendly UMD build
{
input: 'src/main.ts',
output: {
name: 'funnyJoke',
file: pkg.browser,
format: 'umd',
},
},
// CommonJS (for Node) and ES module (for bundlers) build.
// (We could have three entries in the configuration array
// instead of two, but it's quicker to generate multiple
// builds from a single configuration where possible, using
// an array for the `output` option, where we can specify
// `file` and `format` for each target)
{
input: 'src/main.ts',
output: [
{ file: pkg.main, format: 'cjs' },
{ file: pkg.module, format: 'es' },
],
},
]
Add README
Create a README file that explains what your package code is and how to use it.
Test installation locally
You’ll want to test that your package can be imported and used as intended.
You can install a package that’s in your filesystem by running:
npm install path/to/my-package
It makes sense to create another GitHub repo to test your package locally and also to use it to create a CodeSandbox for demo purposes.
Push your latest changes to GitHub
git push
Publish package
npm publish
You might need to login (probably with npm adduser
) first if you encounter any error.
Use published package
If npm publish
ran successfully, then your package should be published now.
Go the NPM website and you should be able to find your package by searching for it or by viewing your packages page:
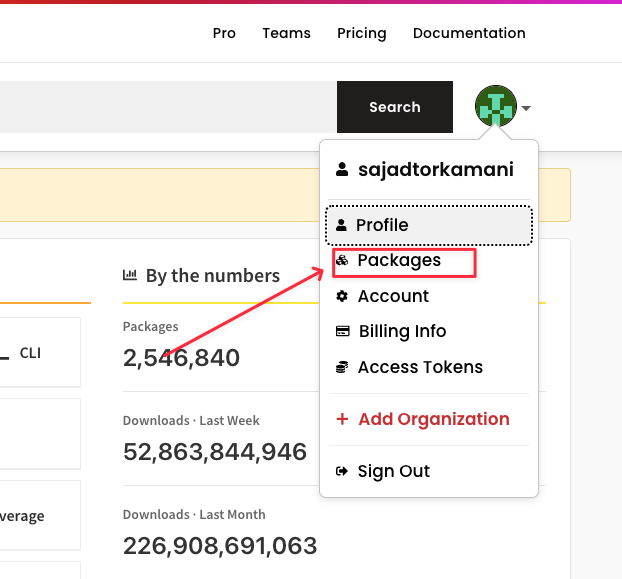
Publish updates
Whenever you make changes, you can increment your NPM version number using:
npm version major|minor|patch
This command will apply the appropriate version change in the version
field of your package.json
file and create a Git commit for it.
Next, you’ll want to run git push
and then npm publish
to publish the version update.
Verify your package has been updated on NPM by running: npm view
.
Sources / further reading
Thanks for your comment 🙏. Once it's approved, it will appear here.
Leave a comment