React rendering process
In a nutshell
- JSX components are compiled into React elements.
- React constructs virtual DOM using your element tree.
- React DOM updates the real DOM to match the virtual DOM (reconciliation).
1. JSX components are compiled into React elements (using React.createElement
).
A tool like Babel compiles your JSX components to React.createElement()
calls. For example, this JSX:
const SimpleComponent = () => (
<div>Hello</div>
)
compiles to:
const SimpleComponent = () => React.createElement("div", null, "Hello")
which in turn creates a React element object like this:
{
type: "div"
key: null
ref: null
props: Object
children: "Hello"
_owner: null
_store: Object
}
React elements are plain JavaScript objects with the following properties:
Property | Description |
type | A string reference to a native DOM element (e.g., span , div , h1 , etc) or to a custom component (e.g., MyCustomComponent ). |
key | Used to uniquely identify an element among siblings (Lists and Keys). |
ref | Reference to a native DOM element. Only defined if you used a ref . |
$$typeof | Something like Symbol(react.element) . See this post for more about prop. |
props | Object of props. Each prop will itself be a React element object with the properties type , key , ref , props , etc. Remember JSX child components will be children prop of a React element. |
2. React constructs virtual DOM using your element tree
When your app first mounts, React calls the render()
method of the root component to construct a tree of React elements. This tree of React elements is known as the virtual DOM.
Because React elements are plain JavaScript objects, it’s cheap and super fast to construct and tear them down compared to interacting with the real DOM. React will recursively walk through your component tree to builds its virtual DOM.
On the initial render, React will mount your component tree on the DOM element you pass to the render
method.
const root = createRoot(container);
root.render(<MyApp />);
On subsequent renders (caused by state updates), it’ll call the component whose state updated trigger the render. React will only re-render the components whose state or props have changed. It won’t re-render the entire element tree.
3. React DOM updates the real DOM to match the virtual DOM (reconciliation).
On initial render, React uses the appendChild()
DOM API to put all the created DOM nodes on the screen.
On the next state or props update of an element tree, the render()
method will return a different tree of React elements.
At this point, there will be two trees:
- Old tree (from the previous render)
- New tree (from the most recent render)
React uses a diffing algorithm to figure out the minimum operations needed to update the existing real DOM (host tree) to reflect the new virtual DOM. Once it has figured out those operations, it applies them to update the real DOM.
As React builds and tears down elements in the tree, it invokes various component lifecycle methods, allowing you to do any setup or clean up work.
Visualisation
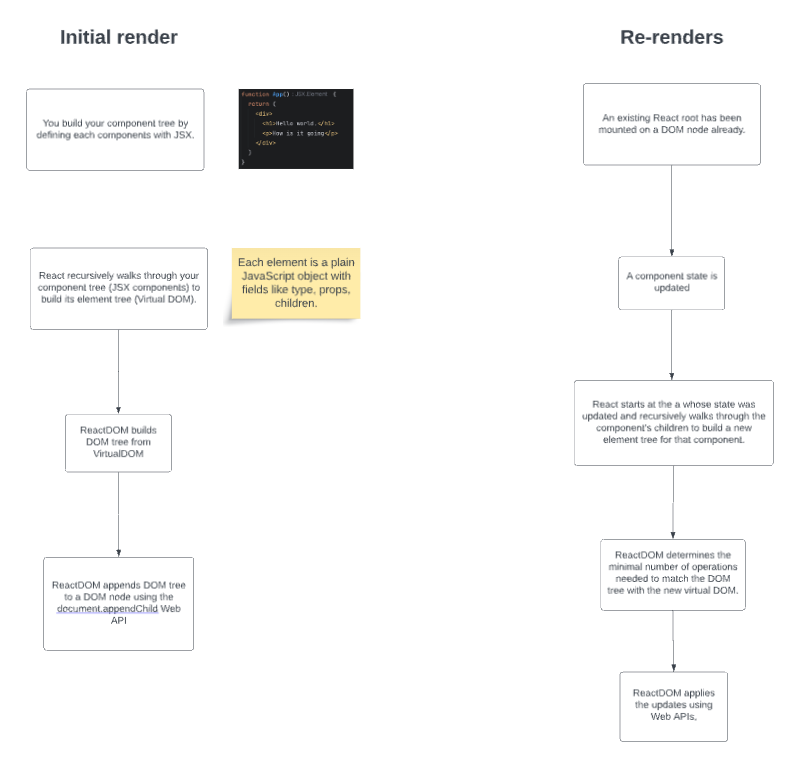
Initial render
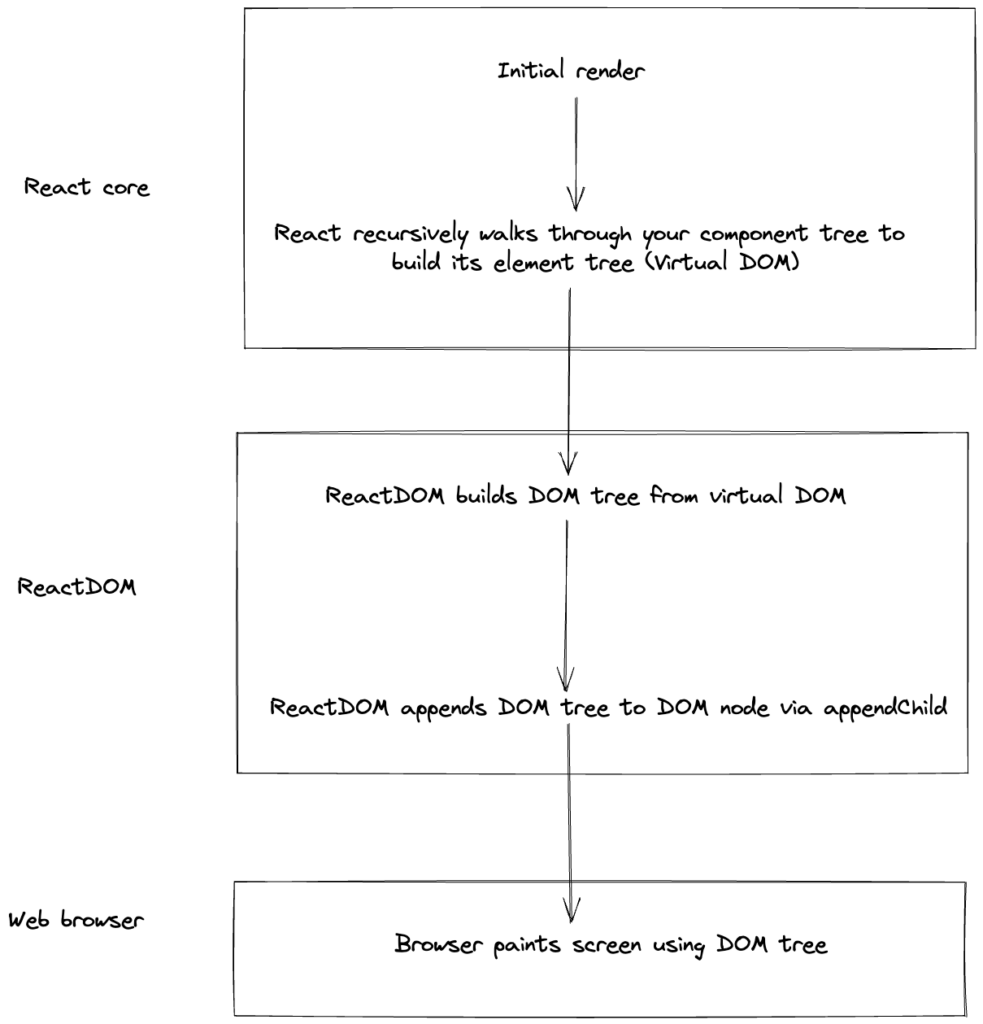
Re-renders
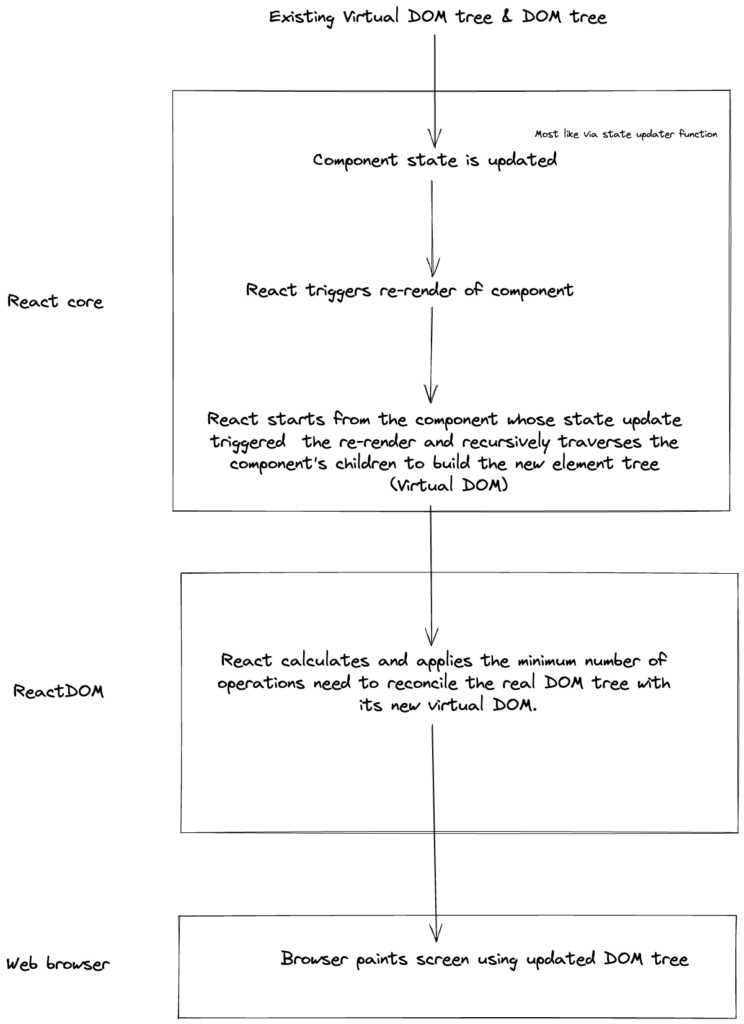
Sources
Thanks for your comment 🙏. Once it's approved, it will appear here.
Leave a comment