SQL left join reference
20 August 2023 (Updated 23 August 2023)
In a nutshell
Left joins let you combine rows from multiple tables. Here’s an example left join:
SELECT a.title, u.name
FROM articles a
LEFT JOIN users u ON u.id = a.user_id
The result of this query will look something like:
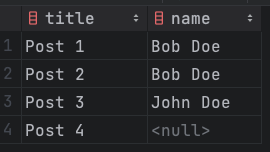
Left joins return null
for any columns (name
in the above example) where there are no matching records from a join table (users
in the above example) are found.
How a left join is processed by a SQL engine
This is what happens when the above query is parsed by a SQL engine:
- The SQL engine begins by selecting a row from the
articles
table (let’s call it “Row A”). - It then performs a
LEFT JOIN
with theusers
table based on the conditionu.id = a.user_id
.- It searches the
users
table for a row where the value ofu.id
(users.id
) is equal to the value ofa.user_id
(articles.id
) from “Row A”. - If a match is found, it retrieves the
name
from the corresponding row in theusers
table (let’s call it “Row U”). - If no match is found, set the result of
name
toNULL
).
- It searches the
- The result set for “Row A” includes the
title
column from “Row A” and the correspondingname
column from “Row U” (if a match was found) orNULL
(if no match was found). - Steps 1-3 are repeated for each row.
What happens when there are multiple matching records in a join table?
Suppose you had the following tables:
users
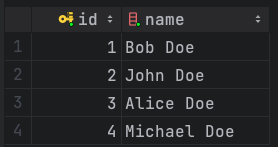
articles
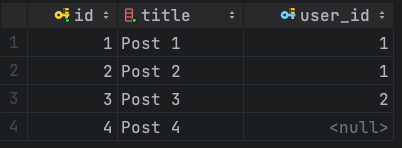
And you executed the following query:
SELECT a.id article_id, u.id user_id
FROM articles a
LEFT JOIN users u ON u.id = a.user_id
The result would look something like:
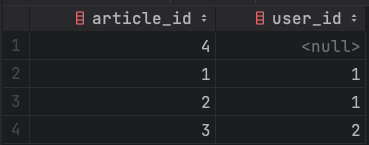
We get two records in the result set where the user_id
is 1
because of how the SQL engine handles multiple matching records in a join table:
- The SQL engine begins by selecting a row from the
articles
table (let’s call it “Row A”).
It then performs aLEFT JOIN
with theusers
table based on the conditionu.id = a.user_id
. - It searches the
users
table for a row where the value ofu.id
(users.id
) is equal to the value ofa.user_id
(articles.id
) from “Row A”. If multiple matches are found as is the case whena.user_id
is1
, then it generates a new row set in the result set. - The result set for “Row A” includes the
id
column from “Row A” and the correspondinguser_id
column from “Row U” (if a match was found) orNULL
(if no match was found).
Steps 1-3 are repeated for each row.
Tagged:
MySQL
Thanks for your comment 🙏. Once it's approved, it will appear here.
Leave a comment