Typescript optional keys reference
7 March 2024 (Updated 7 March 2024)
The problem: Scenarios where optional keys are needed
Consider this UserRecord
:
type UserInfo = 'name' | 'email' | 'age'
type UserRecord = Record<UserInfo, string | number>
This definition makes UserRecord
a type where all the keys (name
, email
, and age
) are required.
So this won’t work:
const partialUser: OptionalUserRecord = {
name: "John Doe" // 'email' and 'age' are optional
}
TypeScript complains with:
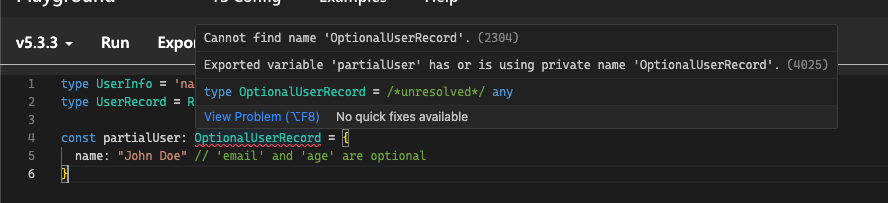
But what if we need a UserRecord
where some keys can be optional?
The solution: Mapped types
We can use mapped types:
type UserInfo = 'name' | 'email' | 'age'
type OptionalUserRecord = {
[K in UserInfo]?: string | number
}
Now, we can do something like:
const partialUser: OptionalUserRecord = {
name: "John Doe" // 'email' and 'age' are optional
}
And TypeScript is happy:
Tagged:
TypeScript
Thanks for your comment 🙏. Once it's approved, it will appear here.
Leave a comment