What is a process?
TLDR: A process is an instance of a computer program that’s being executed by one or more threads.
In a nutshell
A process is an instance of a computer program that’s being executed by the operating system. When the OS executes a program (e.g., node app.js
), it spawns a process that manage that program’s execution.
You can use something like the Activity Monitor on macOS to view information about all the processes running on your machine.
For example, find a process in Activity Monitor:
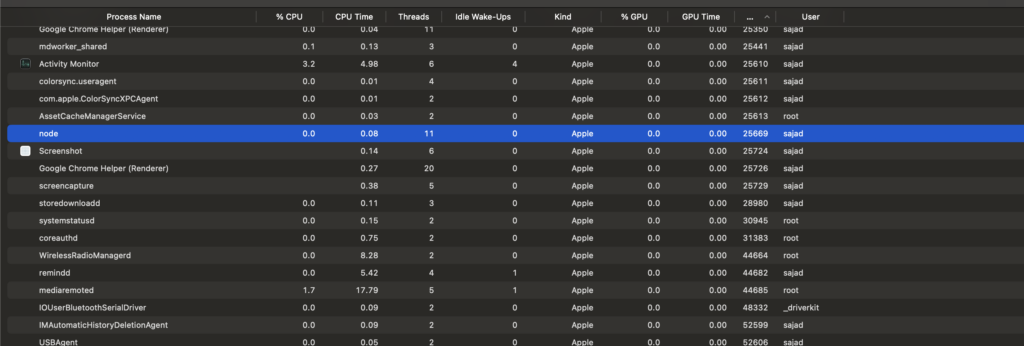
Double-click on the process to view more info:
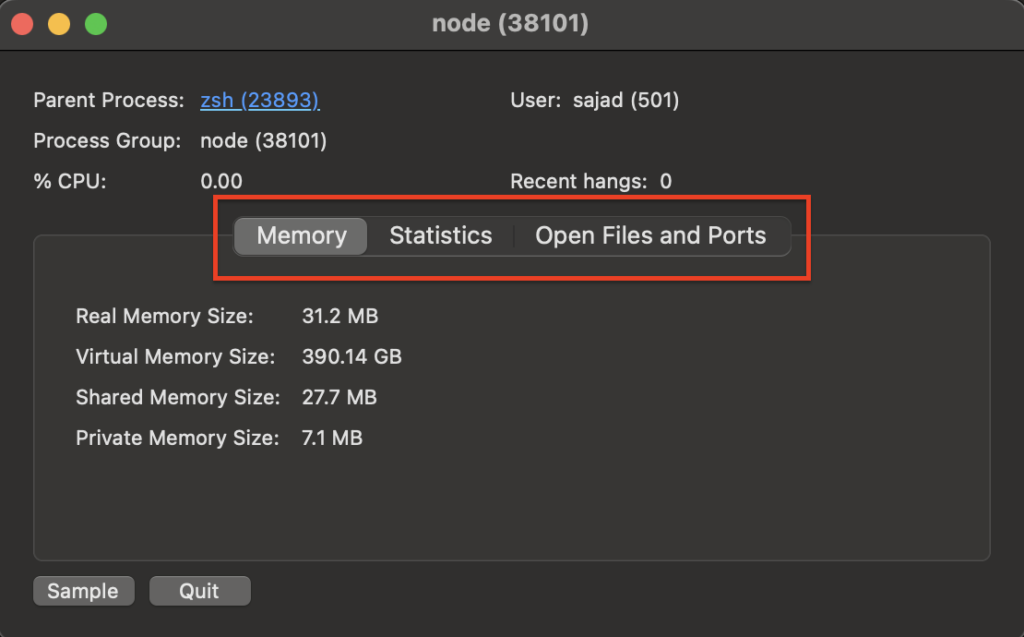
The OS will allocate each resource some data and also hold state information about it:
- Process Control Block (PCB): A data structure that holds information such as process ID, state, priority, and memory allocation,
- Open files: Represents all the files opened by that process for I/O operations. Not accessible to other processes unless explicitly shared.
- System registers: Hardware registers used by the CPU to manage the program’s execution.
Process states
- Ready: Waiting for a CPU to become available.
- Blocked: Waiting for an event to occur (e.g. input or output operation)
- Terminated: Finished executing.
Inter-process communication (IPC)
Each operating system provides inter-process communication (IPC) mechanisms such as:
- Sockets
- Shared memory: multiple processes can read and write from the same memory space.
- Pipes
Spawning an example process using Node.js
For example, if you have a prompt-user.js
file like this:
import * as readline from 'node:readline/promises';
import { stdin as input, stdout as output } from 'node:process';
console.log('---------------------------');
console.log(`PROCESS ID: ${process.pid}`);
console.log('---------------------------');
const rl = readline.createInterface({ input, output });
const answer = await rl.question('What is your name? ');
console.log(`Your name is ${answer}`);
rl.close();
Running node prompt-user.js
will output something like:
❯ node src/process-example/prompt-user.js
---------------------------
PROCESS ID: 24928
---------------------------
What is your name?
You can then use something like htop
to view the details of the process just spawned:

Or the macOS Activity Monitor app:
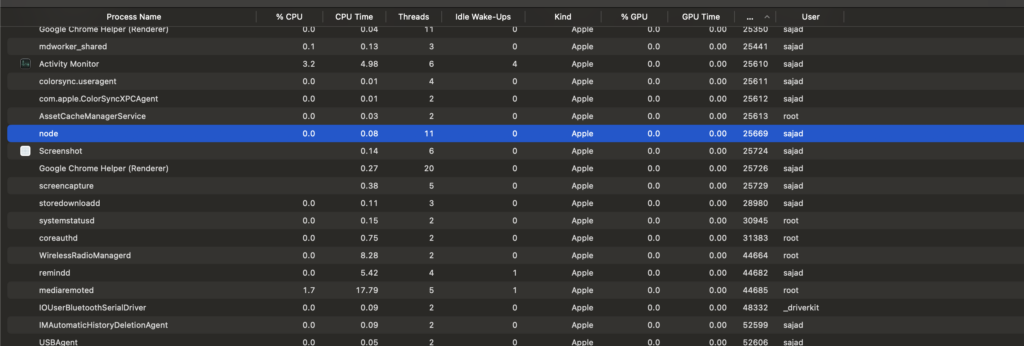
Viewing/managing your processes
You can use something like top
,ps
,htop
for Unix systems, Activity Monitor for macOS, and the Task Manager for Windows to view all the processes running on your machine.
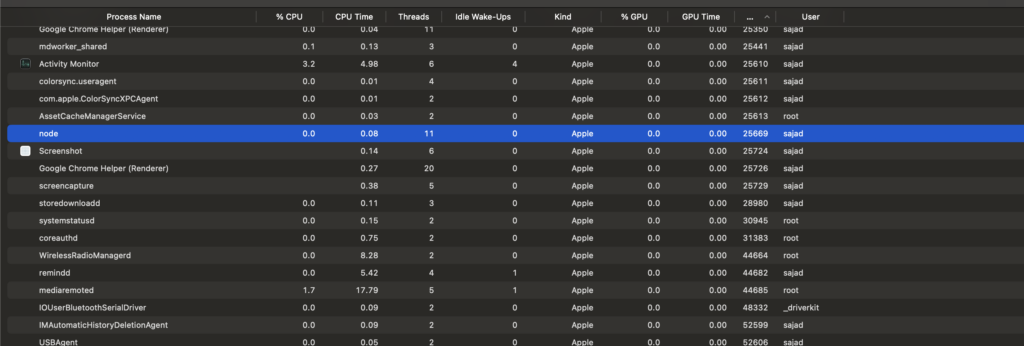
Other notes
- The OS will allocate each process some resources that are private to that process. This provides a measure of isolation from other processes so that if one crashes, others aren’t affected.
Thanks for your comment 🙏. Once it's approved, it will appear here.
Leave a comment